The following are some of the reasons why you would use databases:
•
Compactness: Databases help you maintain large amounts of data and thus completely replace voluminous paper files.
•
Speed: Searches for a particular piece of data or information in a database are
much faster than sorting through piles of paper.
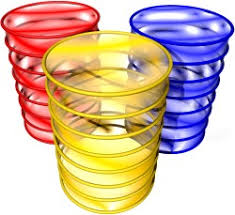
•
Less drudgery: It is a dull work to maintain files by hand; using a database completely eliminates such maintenance.
•
Currency: Database systems can easily be updated and so provide accurate information all the time and on demand.
Benefits of Using a RelationalDatabase Management System
RDBMSs offer various benefits by controlling the following:
•
Redundancy: RDBMSs prevent you from having duplicate copies of the same data,
which takes up disk space unnecessarily.
•
Inconsistency: Each redundant set of data may no longer agree with other sets of
the same data. When an RDBMS removes redundancy, inconsistency cannot
occur.
•
Data integrity: Data values stored in the database must satisfy certain types of
consistency constraints.
•
Data atomicity: In event of a failure, data is restored to the consistent state it
existed in prior to the failure. For example, fund transfer activity must be atomic.
•
Access anomalies: RDBMSs prevent more than one user from updating the same
data simultaneously; such concurrent updates may result in inconsistent data.
•
Data security: Not every user of the database system should be able to access all
the data. Security refers to the protection of data against any unauthorized access.
•
Transaction processing: A transaction is a sequence of database operations that
represents a logical unit of work. In RDBMSs, a transaction either commits all the
changes or rolls back all the actions performed until the point at which the failure
occurred.
•
Recovery: Recovery features ensure that data is reorganized into a consistent state
after a transaction fails.
•
Storage management: RDBMSs provide a mechanism for data storage
management. The internal schema defines how data should be stored.
Comparing Desktop and Server RDBMS Systems
In the industry today, you’ll mainly work with two types of databases: desktop databases and server
databases
Desktop Databases
Desktop databases are designed to serve a limited number of users and run on desktop PCs, and they
offer a less-expansive solution wherever a database is required. Chances are you have worked with a
desktop database program; Microsoft SQL Server Express, Microsoft Access, Microsoft FoxPro,
FileMaker Pro, Paradox, and Lotus are all desktop database solutions.
Desktop databases differ from server databases in the following ways:
•
Less expensive: Most desktop solutions are available for just a few hundred dollars.
In fact, if you own a licensed version of Microsoft Office Professional, you’re
already a licensed owner of Microsoft Access, which is one of the most commonly
and widely used desktop database programs around.
•
User friendly: Desktop databases are quite user friendly and easy to work with,
because they do not require complex SQL queries to perform database operations
(although some desktop databases also support SQL syntax if you want to write
code). Desktop databases generally offer an easy-to-use graphical user interface.
Server Databases
Server databases are specifically designed to serve multiple users at a time and offer features that allow
you to manage large amounts of data very efficiently by serving multiple user requests simultaneously.
Well-known examples of server databases include Microsoft SQL Server, Oracle, Sybase, and DB2.
The following are some other characteristics that differentiate server databases from their desktop
counterparts:
•
Flexibility: Server databases are designed to be very flexible and support multiple
platforms, respond to requests coming from multiple database users, and perform
any database management task with optimum speed.
•
Availability: Server databases are intended for enterprises, so they need to be
available 24/7. To be available all the time, server databases come with some highavailability features, such as mirroring and log shipping.
•
Performance: Server databases usually have huge hardware support, so servers
running these databases have large amountsof RAM and multiple CPUs. This is
why server databases support rich infrastructure and give optimum performance.
•
Scalability: This property allows a server database to expand its ability to process
and store records even if it has grown tremendously.